When embarking on the journey of building a React Native app, scalability is a crucial consideration. As your app grows in features, users, and complexity, maintaining performance and codebase manageability becomes paramount. In this guide, we will explore the key aspects of building a scalable React Native app, from project structure to deployment strategies.
1. Project Structure: Organize for Clarity and Maintenance
Organize Codebase
Structuring your React Native codebase is crucial for long-term maintainability. Consider adopting a modular folder structure, grouping related files and components together based on features rather than file types. This not only makes the codebase more organized but also eases navigation and collaboration among developers.
Creating clear distinctions between components, containers, services, and other parts of your app allows for easier maintenance and updates. A well-organized codebase simplifies onboarding for new developers and makes it more straightforward to locate and understand specific functionalities.
Separation of Concerns
Separating concerns within your React Native app involves keeping distinct parts of your application focused on specific tasks. For instance:
Business Logic and UI Components:
Keep business logic separate from UI components. This is commonly achieved by using container components to manage state and business logic, while presentational components focus solely on rendering UI elements.
Modularity:
Divide your app into smaller, manageable modules based on features. Each module should encapsulate related components, services, and logic.
2. State Management: Efficiently Handle Data
Redux
Redux is a predictable state container for JavaScript apps, and it can significantly aid in state management for React Native applications. In a scalable React Native app:
Reducers and Actions:
Organize reducers and actions logically. Group related actions and reducers together, making it easier to maintain and expand your state management as your app grows.
Middleware for Asynchronous Operations:
Use middleware like Redux Thunk to handle asynchronous operations. This ensures that your state management seamlessly integrates with asynchronous tasks such as API calls.
Redux Toolkit
Redux Toolkit is a set of tools and guidelines that simplifies working with Redux. It promotes best practices and reduces boilerplate code:
Slice:
Utilize slices to logically group together reducers and actions. Slices encapsulate a piece of the Redux state and its associated logic.
CreateAsyncThunk:
For handling asynchronous operations, leverage createAsyncThunk. It streamlines the process of dispatching actions and managing loading, success, and error states.
3. Networking: Optimize API Calls for Efficiency
Implement Efficient Data Fetching
Efficiently fetching data is crucial for the performance and responsiveness of your React Native app. Consider the following:
Pagination:
Implement pagination for large datasets. Fetching only the necessary data at a time reduces the load on your network and improves the user experience.
Lazy Loading:
Adopt lazy loading to load data on-demand. This is especially useful for optimizing the loading of images, videos, or other assets.
Error Handling:
Implement robust error handling for API calls. Provide meaningful feedback to users in case of errors and handle edge cases gracefully to prevent app crashes.
4. Performance Optimization: Enhance User Experience
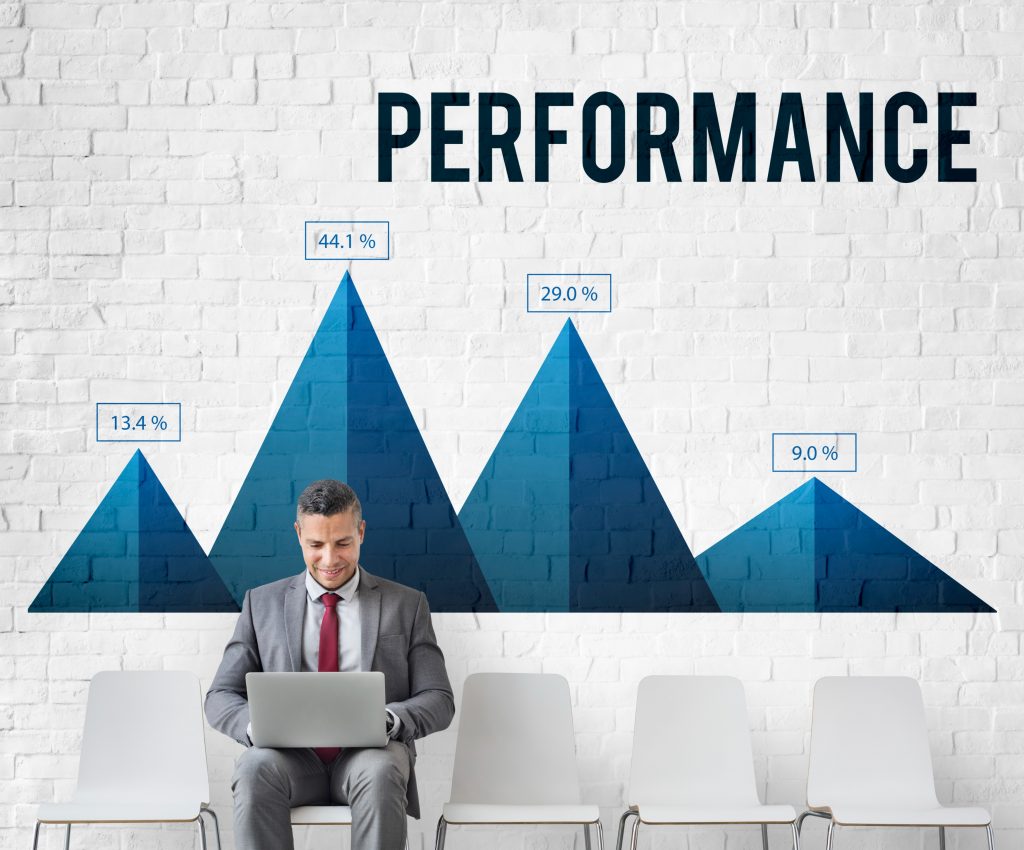
Code Splitting
Code splitting involves breaking down your app’s code into smaller chunks that are loaded as needed. This optimization technique is vital for enhancing the performance of your React Native app:
Dynamic Imports:
Use dynamic imports to load modules on demand. This is particularly useful for larger applications where loading the entire codebase at once might impact the app’s initial load time.
Optimized Rendering:
Consider using the React Navigation library for efficient navigation and screen rendering. React Navigation allows you to lazily load screens, optimizing the rendering process.
Virtualized Lists
In React Native, lists are a common UI component. Virtualized lists render only the items currently visible on the screen, making them an excellent choice for handling large datasets:
Performance Benefits:
Virtualized lists significantly improve the performance of rendering long lists by only rendering the items in the viewport, preventing unnecessary rendering of off-screen elements.
Libraries:
Explore libraries such as react-virtualized or react-window for implementing virtualized lists in your React Native app.
5. Scalable UI: Design for Various Screen Sizes
Responsive Design
Creating a responsive UI is essential for providing a consistent user experience across different devices and screen sizes:
Flexbox Layouts:
Utilize Flexbox for creating flexible layouts that adapt to various screen sizes. Flexbox is a powerful layout system that allows you to design responsive and dynamic UIs.
Media Queries:
Implement media queries in your stylesheets to apply specific styles based on the device’s characteristics, such as screen width.
UI Libraries
Leverage UI component libraries to maintain a consistent and visually appealing design:
Material-UI or Ant Design:
Consider using popular UI libraries like Material-UI or Ant Design, which provide pre-designed and well-tested components. These libraries save development time and ensure a cohesive design language throughout your app.
6. Testing: Ensure Reliability and Maintainability
Unit Testing
Unit testing involves testing individual units or components of your React Native app in isolation. This ensures that each part of your application works as expected:
Critical Functions:
Identify critical functions and components that form the backbone of your app. Write unit tests to verify their correctness.
Jest:
Jest is a widely-used testing library for JavaScript applications, including React Native. It provides a robust framework for writing and running unit tests.
Integration Testing
Integration testing focuses on how different components work together as a whole:
User Interactions:
Simulate user interactions and test the flow of data between various components.
React Testing Library:
Use React Testing Library to create tests that interact with your app as a user would. This ensures that your components integrate seamlessly.
7. Security: Safeguard Your App and User Data
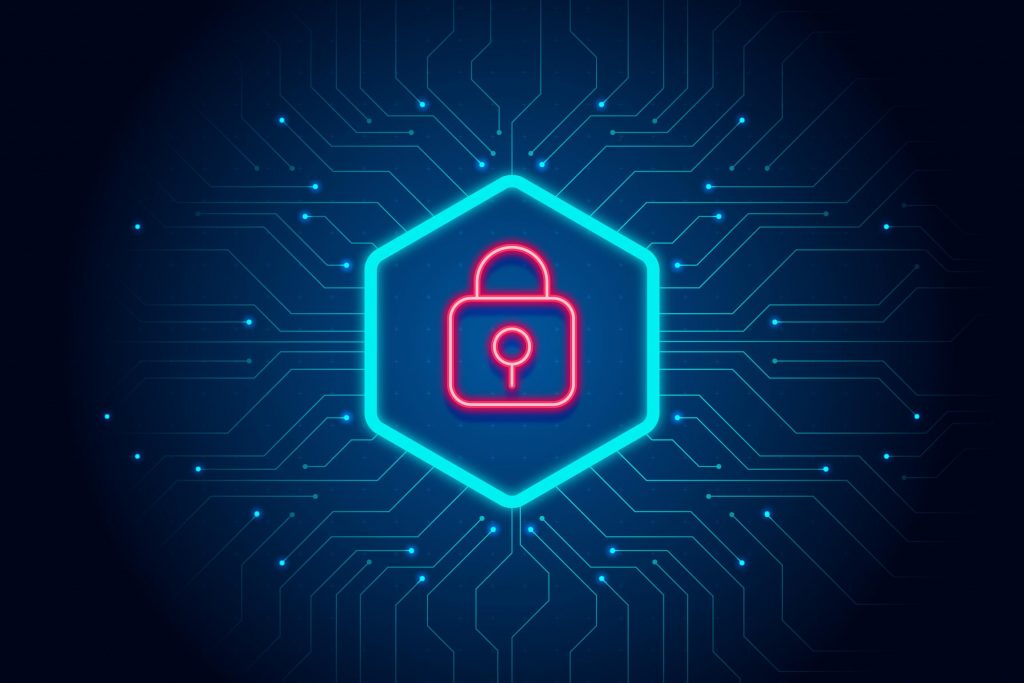
Secure Storage
Ensuring the security of your React Native app involves protecting sensitive data, such as user credentials and authentication tokens:
Keychain/Keystore:
Utilize the device’s secure storage mechanisms like Keychain on iOS or Keystore on Android to securely store sensitive information.
Token Security:
Implement secure practices for token-based authentication, such as using JSON Web Tokens (JWTs) and ensuring they are transmitted over secure channels.
Network Security
Secure communication with APIs is vital to prevent unauthorized access or data breaches:
HTTPS:
Always use HTTPS for communication between your app and APIs to encrypt data in transit.
Security Audits:
Regularly conduct security audits and address any identified vulnerabilities promptly.
8. Continuous Integration/Continuous Deployment (CI/CD): Automate Your Workflow
Automated Builds
Continuous Integration (CI) and Continuous Deployment (CD) streamline your development workflow by automating various processes:
Automated Testing:
Set up CI pipelines to run automated tests whenever changes are pushed to the repository. This ensures that new code doesn’t introduce regressions.
Deployment Automation:
Implement CD pipelines to automate the deployment process. This reduces the chance of deployment errors and ensures a consistent deployment process.
Jenkins, CircleCI, GitHub Actions:
Popular CI/CD tools like Jenkins, CircleCI, and GitHub Actions can be configured to automate your build and deployment workflows.
9. Monitoring and Analytics: Track Performance and User Behavior
Error Tracking
Identifying and resolving errors quickly is crucial for maintaining a stable React Native app:
Sentry, Bugsnag:
Integrate error tracking tools like Sentry or Bugsnag to receive real-time notifications of errors occurring in your app.
Logging:
Implement comprehensive logging to capture relevant information about errors, making it easier to diagnose and fix issues.
Analytics
Understanding user behavior is essential for making informed decisions and improving your app:
Google Analytics, Mixpanel:
Integrate analytics tools such as Google Analytics or Mixpanel to track user interactions, navigation patterns, and other key metrics.
Custom Events:
Implement custom events to track specific user actions within your app. This data can inform feature enhancements and optimizations.
10. Documentation: Keep Your Codebase Understandable
Code Documentation
Thorough documentation is essential for making your codebase understandable and maintainable:
JSDoc:
Use JSDoc comments to document your JavaScript code. This includes providing descriptions for functions, parameters, and return values.
Inline Comments:
Add inline comments to clarify complex sections of code or to provide context for future developers.
Readme and Guides
Comprehensive project documentation facilitates collaboration and onboarding:
Readme:
Create a detailed Readme file that includes information about the project structure, installation instructions, and how to contribute.
Contributing Guidelines:
Provide contributing guidelines to help new developers understand the development workflow, coding standards, and how to submit contributions.
In Summation: Navigating React Native Scalability
In conclusion, building a scalable React Native app requires a comprehensive approach, addressing aspects from code organization to deployment strategies. By following these best practices, you can develop an application that not only meets current needs but also positions itself for future growth and scalability challenges.
For expert guidance and tailored solutions, consider reaching out to GeekyAnts—an industry leader with a proven track record in React Native development. Should you have any further questions or need additional assistance, please feel free to contact us.